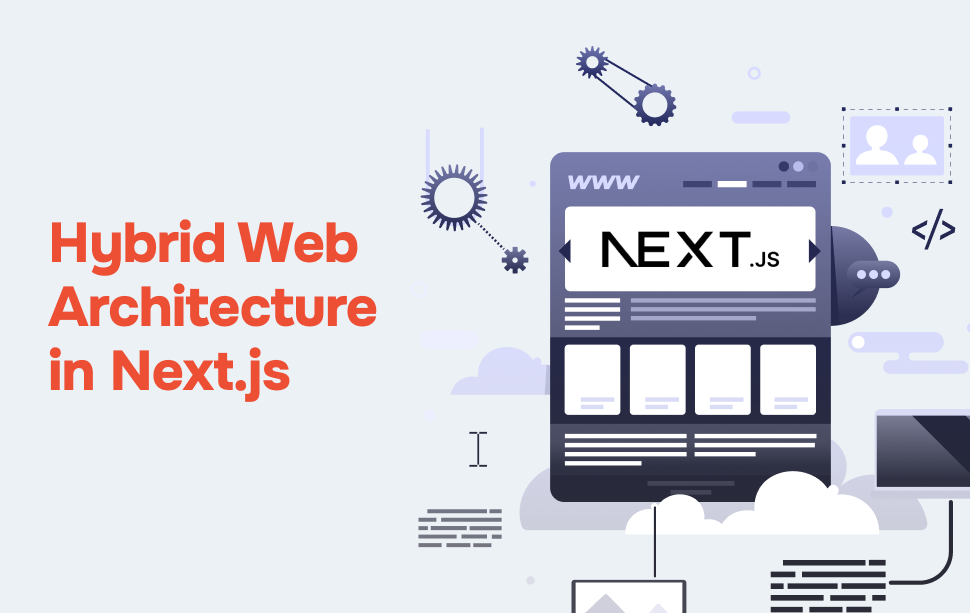
Brief Overview of Next.js
Next.js is a popular open-source React framework created by Vercel, which is known for its hybrid approach to rendering, as well as a number of other features that make it an excellent choice for many web development projects. It allows for server-side rendering (SSR), static site generation (SSG), and client-side rendering (CSR), providing an optimal user experience through fast page loads and a better user experience. Next.js has been designed for gradual adoption, which means you can continue to use your existing code and add as much (or as little) React as you need.
Introduction to the Concepts of ISR, SSR, and SSG
There are three main rendering strategies in Next.js: Server-Side Rendering (SSR), Static Site Generation (SSG), and Incremental Static Regeneration (ISR).
- Server-Side Rendering (SSR) is the process of rendering web pages on the server as opposed to the client. This means that the server generates the necessary HTML for a page before it is sent to the client.
- Static Site Generation (SSG) is a process where the server generates a full static HTML page at build time, which can then be distributed to various CDNs for faster access.
- Incremental Static Regeneration (ISR) is a rendering method that allows you to update static content after it’s been generated, combining the benefits of SSG and SSR. It allows static content to be updated over time, rather than requiring a full rebuild of the site like in traditional SSG.
Server Side Rendering (SSR)
A. Definition and Explanation of SSR
Server-Side Rendering (SSR) refers to the process where a web server generates the full HTML for a page in response to a user request, then sends the fully rendered page to the client. With SSR, the server does most of the work: it executes the necessary JavaScript code, retrieves data from APIs, performs computations, and renders the page as complete HTML content.
B. When to Use SSR
There are a few scenarios where SSR can be particularly beneficial:
1. SEO Benefits
Because SSR results in a fully rendered page, search engines can easily crawl and index your site. This leads to better search engine rankings and visibility.
2. Initial Load Performance
SSR also improves the initial load performance of your site. Pre-rendered HTML is sent from the server, resulting in shorter load times and a better user experience, especially on slower network connections or less powerful devices.
C. How to Implement SSR in Next.js
Implementing SSR in Next.js involves the use of a specific Next.js function: getServerSideProps.
1. Using getServerSideProps
In Next.js, you can use the getServerSideProps function to fetch data on each request. This function runs on the server-side for every request and the output of it is the props used to generate the page.
2. Example Code Snippet
Here’s a basic example of implementing SSR in Next.js:
In this example, the getServerSideProps function is a special function in Next.js that runs on the server for every request. It fetches data and passes it as props to the HomePage component, which is then rendered on the server and sent to the client.
D. Advantages and Disadvantages of SSR
While SSR offers many benefits, there are also some drawbacks to be aware of:
Advantages:
- Improved SEO: As SSR delivers a fully rendered page to the client, search engines can easily crawl and index these pages, leading to better search engine rankings.
- Fast Initial Load: SSR sends a fully rendered page to the client, resulting in faster initial page loads and a better user experience.
- Real-time data: SSR is ideal for displaying data that is constantly changing in real-time, as it generates the HTML for each request.
Disadvantages:
- Server Load: As SSR generates a new HTML document with each request, it can put a significant load on the server, especially with heavy traffic.
- Time To First Byte (TTFB): The time to first byte (TTFB) can be slower with SSR compared to SSG or ISR, as the server needs to generate the HTML document.
Static Site Generation (SSG)
A. Definition and Explanation of SSG
Static Site Generation (SSG) is a method of rendering where static HTML files are generated at build time. This means that the HTML files are generated once and then served to the client for each request. As the pages are pre-rendered and stored, they can be delivered to the user incredibly quickly.
B. When to Use SSG
Static Site Generation is most beneficial in the following scenarios:
1. Performance Benefits
SSG leads to very fast loading times, which improves the user experience and can also help your website’s SEO since search engines favor fast and responsive websites that are quick for users to interact with.
2. Security Benefits
As the pages are pre-rendered and there’s no server-side processing involved in serving the pages, there’s less surface for attacks, which makes SSG sites more secure.
C. How to Implement SSG in Next.js
Implementing SSG in Next.js involves the use of a specific Next.js function: getStaticProps.
1. Using getStaticProps
In Next.js, you can use the getStaticProps function to fetch data at build time. This function runs on the server-side once during the build process, and the output of it is the props used to generate the page.
2. Example Code Snippet
Below is a basic example of implementing SSG in Next.js:
In this example, the getStaticProps function fetches data from an API at build time. The fetched data is then passed as props to the HomePage component, which is then rendered as static HTML files.
D. Advantages and Disadvantages of SSG
While SSG has many advantages, it also has some potential drawbacks:
Advantages:
- High Performance: As pages are pre-rendered and served as static HTML files, SSG results in very fast load times.
- SEO Friendly: As with SSR, SSG delivers fully rendered pages that can be easily crawled and indexed by search engines.
- Enhanced Security: Since there’s no server-side processing involved in serving the pages, SSG sites are more secure.
Disadvantages:
- Not Suitable for Dynamic Content: Since pages are generated at build time, SSG is not suitable for pages that need to display real-time or frequently changing data.
- Build Time: If a site has a large number of pages, the build time can be quite long.
Incremental Static Regeneration (ISR)
A. Definition and Explanation of ISR
Incremental Static Regeneration (ISR) is a feature introduced in Next.js that allows you to create static pages that are updated incrementally after they’ve been generated, combining the benefits of SSG and SSR. ISR allows static content to be updated over time, rather than needing a full rebuild of the site like in traditional SSG.
B. When to Use ISR
ISR is best used in the following scenarios:
1. For Dynamic Content
ISR is an excellent choice for sites that display somewhat dynamic data but don’t need to be updated with every single request. With ISR, your users get the speed of a static site with the flexibility of server-rendered content, making it perfect for pages that need to display somewhat dynamic data.
2. For a Balance Between SSR and SSG
ISR offers a good balance between the benefits of SSR and SSG. It allows you to serve static content that updates incrementally as needed, providing a much more scalable solution for sites with dynamic content.
C. How to Implement ISR in Next.js
Implementing ISR in Next.js is straightforward because of the getStaticProps function and the revalidate property.
1. Using getStaticProps with revalidate
The getStaticProps function is used to fetch the data needed for pre-rendering, and the revalidate property specifies how often (in seconds) a page revalidation should occur.
2. Example Code Snippet
D. Advantages and Disadvantages of ISR
Like with other methods, ISR also has its own set of advantages and drawbacks:
Advantages:
- Balances Static and Dynamic Content: ISR allows for static content to be served quickly while still being able to update the content as needed.
- Scalability: ISR provides a more scalable solution for sites with large amounts of dynamic content. </li?
Disadvantages:
- Delay in Updates: There can be a delay in updates being visible to users as the page is updated in the background.
- Not Suitable for Real-Time Data: If your site needs to display real-time data, ISR might not be the best choice as there can be a delay in the data being updated on the page.
Comparison of SSR, SSG, and ISR
A. When to Use Each Method
Choosing between SSR, SSG, and ISR depends on the specific needs of your application:
1. SSR for Dynamic, User-Specific Content
If your site needs to display data that changes frequently or is specific to individual users, SSR is the way to go. It ensures that the data is always fresh and up-to-date for each request.
2. SSG for Static, Unchanging Content
If your site has pages with content that doesn’t change frequently, SSG is ideal. As pages are pre-rendered and served as static HTML files, SSG results in very fast load times.
3. ISR for a Balance of Dynamic and Static Content
If your site has pages that need to display somewhat dynamic data, ISR is an excellent choice. It allows for static content to be served quickly while still being able to update the content as needed.
B. Performance Comparison
In terms of performance, SSG typically outperforms both SSR and ISR. This is because SSG pages are pre-rendered and served as static HTML files, resulting in very fast load times. However, SSR and ISR can still deliver good performance, especially when implemented properly with consideration for caching and other optimization techniques.
C. SEO Implications
SSR and SSG both deliver fully rendered pages that can be easily crawled and indexed by search engines, leading to better SEO. ISR also provides SEO benefits as the statically generated pages can be indexed by search engines. However, the SEO benefits of ISR can be slightly less than SSR and SSG due to the potential delay in updates being visible to users.
D. Use Cases and Examples
Here are some real-life examples and use cases where each rendering method might be preferred:
- SSR – E-commerce Sites: E-commerce sites often need to display real-time data like stock levels and prices. SSR is a good choice in this case as it ensures the latest data is displayed to the user.
- SSG – Blogs and Marketing Pages: Blogs and marketing pages that have largely static content can benefit from SSG as it leads to very fast load times.
- ISR – News Websites: News websites often need to display somewhat dynamic content but don’t need to be updated with every single request. In this case, ISR is a great option as it allows static content to be served quickly while still being able to update the content as needed.
Are you considering a hybrid web architecture?
Hexon Global has a decade-long track record of assisting companies in navigating hybrid web architecture and cloud challenges. If you would like us to identify tailored solutions for your business, reach out to us
Contact Us
Get in touch
Understand how we help our clients in various situations and how we can be of service to you!